The obniz cloud API is an API for manipulating devices tied to an account, serverless events, etc. You can use it for yourself or other users by creating a WebApp and getting approval from the user. Currently, the following information can be manipulated
- WebApp itself
- WebApp installation information
- User information for approved users
- Device owned by the authorized user
- Serverless events for approved users
GraphQL API
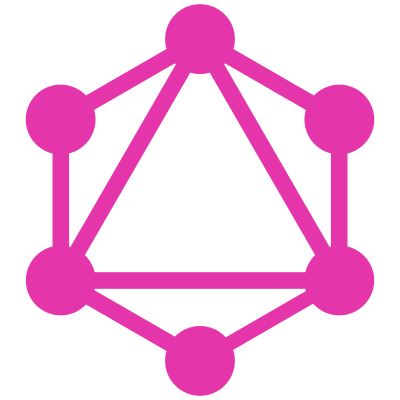
The obniz cloud API employs GraphQL. The endpoint is below
https://api.obniz.com/v1/graphql
You can get the results by giving a token and query to the endpoint.
const graphQLClient = new GraphQLClient(`https://api.obniz.com/v1/graphql`, {
headers: {
authorization: `Bearer ${token}`,
},
});
const query = `{
user {
id,
name,
email
}
}`;
const user = await graphQLClient.request(query);
GraphQL communication is done using the HTTPS protocol.
Therefore, even if you cannot use a GraphQL client, you can handle it as you would a normal HTTP request.
For details, please refer to here.
About GraphQL
GraphQL is a query language that allows you to retrieve only the data you need, similar to the SELECT statement in SQL.
There are two types of GraphQL API, Query and Mutation, and they can be handled as follows.
- Query ≒ SELECT statement
- Mutation ≒ UPDATE statement
For details, please refer to here.
Document
https://api.obniz.com/v1/graphql
in your browser, you can open the API demo screen along with the documentation.
To use the API, you need to add a token to the header, so it cannot be used in the online demo screen.
It is convenient to make use of the GraphQL application for the desktop that can give a token to a request header for actual use.
SDK
TypeScript/JavaScript SDK for using GraphQL endpoint is available.
https://github.com/obniz/obniz-cloud-sdk
Since it is type-defined, you can use IDE completion and TypeScript type checking.
Please refer to README for usage.
Token
In order to use the API and access user information, you must create a WebApp and have the user approve the WebApp. A token will be issued upon approval. Also, there are two types of tokens: those issued per user, and those for the WebApp itself.
- WebApp token: A token for the WebApp itself. You can get the installation information.
- OAuth token: A token for each user that is obtained when the user approves. Information tied to the user can be manipulated.
WebApp is required to obtain each. Please see the App for more information.
A token is given in the request header when tapping the GraphQL API.
authorization: Bearer oauth_xxxxxxx
Sample code
There is a sample code on GitHub that uses the API.
Using OAuth to manipulate user and device information
https://github.com/obniz/obniz-webapp-example-oauth
How to use installation to obtain user-installed application information and develop web services
https://github.com/obniz/obniz-webapp-example-install
Install
In IoT development, there are scenes where the same program is used across multiple devices, and each device runs with different settings. With obniz's WebApp, users not only approve and issue access tokens, but they can also "install" multiple WebApps with their settings.
The user installation process is as follows.
- The server system runs on AWS and other cloud services and is registered as a WebApp on the obniz cloud.
- Users approve WebApps
- User installs a WebApp
During installation, the user is asked about the settings provided in the WebApp and they are saved during the installation.
Also, if a Webhook URL is set in the WebApp, a webhook will be issued upon installation. - WebApp calls the obniz cloud API to retrieve all installed WebApps.
Execute the program based on the installation information in the WebApp, and in some cases, auto-scale out. - Synchronize the WebApp with the user's configuration via a webhook issued at each user installation event (creating/updating/deleting an installation) or a periodic fetch
To learn more about installing the WebApp, please see below.
Error code list
Code | Description | Solution |
---|---|---|
PERMISSION_DENIED | You do not have sufficient access privileges. | Grant the necessary permissions to the API key. |
UNAUTHORIZED | Authentication is required. | Please put the token in the Authorization header. |
BAD_TOKEN_TYPE | Token type is mismatched to one this API requires. | Match the API and token type, e.g. use app tokens for app-related APIs. |
INTERNAL_SERVER_ERROR | An unexpected server error has been occurred. | This is an error on the obniz Cloud side, so please wait a while and it may be resolved. |
BAD_REQUEST | Validation rejected. | Make sure you have not entered incorrect values, such as unusable characters. |
TOO_MANY_REQUESTS | Too many requests. | Please take some time and request again. |
Code | Description |
---|---|
OBNIZ_NOT_FOUND | obniz was not found. |
OBNIZ_INVALID_REGISTRATE_URL | Invalid URL to registrate an obniz. |
OBNIZ_INVALID_SERIAL_CODE | Invalid serial code. |
OBNIZ_INVALID_TOKEN | Invalid registration token. |
OBNIZ_INVALID_ID | Invalid obniz id. |
OBNIZ_INSTALLED_APP | App has already been installed on obniz. |
OBNIZ_ALREADY_OWN | You already own this obniz. |
OBNIZ_UNREGISTERED | This obniz has not been registered. |
OBNIZ_DELETED_ACCESS_TOKEN | The access token has already been deleted. |
OBNIZ_GENERATED_ACCESS_TOKEN | The access token has already been generated. |
OBNIZ_NOT_INSTALLED_APP | App is not installed on this obniz. |
OBNIZ_OWNED_BY_ANOTHER_USER | This obniz is owned by an another user. |
OBNIZ_INACTIVE | This obniz is inactive. |
OBNIZ_MISMATCH_INSTALLED_APP | An app you specified with your request and one that is installed on this obniz must be the same. |
APP_NOT_FOUND | App was not found. |
APP_INVALID_ID | Invalid App id. |
USER_NOT_FOUND | User was not found. |
USER_INVALID_ID | Invalid user id. |
FACILITY_NOT_FOUND | Facility does not exist. |
FACILITY_INVALID_ID | Invalid facility id. |
EVENT_NOT_FOUND | Event was not found. |
EVENT_INVALID_ID | Invalid event id. |
HARDWARE_NOT_FOUND | Hardware was not found. |
FACILITY_OPERATION_NOT_FOUND | Facility operation was not found. |
FACILITY_OPERATION_INVALID_ID | Invalid facility operation id. |
FACILITY_OPERATION_ALREADY_EXIST_ID | Facility operation id already exists. |
TEAM_NOT_ALLOWED_SET_PASSWORD | Team account can not set password. |
SEARCH_OVER_LIMITATION_DEVICE_COUNT | Too many search results. |
SERIALCODE_NOT_FOUND | Serial code was not found. |